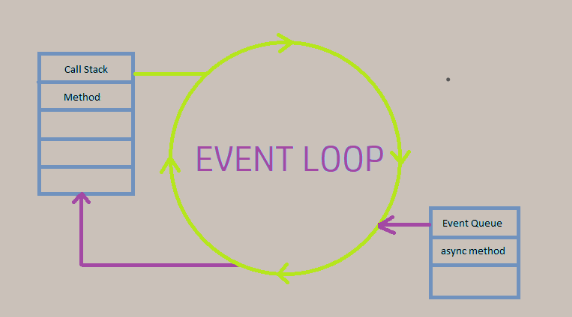
What is an event loop?
What is the event loop inside javascript? Being a javascript engineer, I find it utterly humorous I only found out about this after so many months. So here is your chance to redeem yourself. It is never too late to learn something! This article is curated with reference to the youtube video you can find at the bottom of this page. He is an awesome speaker; eloquent and clear.
In Javascript, we do hear this term called the V8 Engine. It is used as during Chrome’s runtime but what exactly is it? You can find out more here. But here’s a short description of that:
V8 is Google’s open source high-performance JavaScript and WebAssembly engine, written in C++.
As it is widely known, Javascript is basically a single-threaded non-blocking asynchronous concurrent language. Javascript have a call stack, an event loop, a callback queue and some other apis. Lets dive in to understand what are the different components.
In this article, we will just briefly go through 3 key components
1. What are the different components of a javascript environment
Briefly, what is the v8 engine? Every thing you see around you are a composition of many other smaller things. Take the example of a guitar. There are many components: strings, wood, etc. Each of these components plays their individual roles. In the same way, JS environment is beautifully made by its components.
2. What is the call stack
we will go through what exactly does it do? An example to help us to understand. And what is the common error when a function is called recursively (a function calling itself). A stack of cards. One piece on top of another, we can better understand the role and implementation of a JS callstack with this analogy.
3. What is an event loop
What is its definition? What happens when we call a setTimeout function? What happens when we actually call a function with setTimout, t=0? We will find out how how the event loop handles such an implementation. I know of a really interesting beatboxer video and at 7:40mins, it goes: Loop loop loop loop loop. Describing just what an event loop does. Haha
#1. What are the different components of a javascript environment?
Fundamentally, from the most basic level of understanding JavaScript (I know that are a whole lot more components behind the JavaScript engine & environment), there are 3 key components in the JavaScript environment. The first component will be the Memory heap and Call stack. This is where the Chrome V8 engine resides in as well. Here’s a brief description of what the V8 engine does.
V8 compiles and executes JavaScript source code, handles memory allocation for objects, and garbage collects objects it no longer needs. V8’s stop-the-world, generational, accurate garbage collector is one of the keys to V8’s performance
Call stack is used for static memory allocation and Heap for dynamic memory allocation, both stored in the computer’s RAM. Did some research into the differences and it is way more technical than I expected it to be; which feels like one going down a rabbit hole. Will definitely savour this knowledge another time!
For now, a basic understanding that heap is used for memory allocation of the variables created in your function. And stack is used for the execution of functions that are called. If you wish to find out more, i’ve found a really insightful medium article talking about it. You can check them out here

Second component is the WebAPIs component. Here is where your browser ajax functions and DOM components actually reside in. Generally Application Programming Interfaces (APIs) are constructs made available in programming languages to allow developers to create complex functionality more easily. It is a form of abstraction to keep complex code away from the user, providing easier syntax to be used in place of it.

A fun fact about Nodejs: If you are aware of nodejs being a javascript language, it basically has similar components but swapping out the WebAPIs with C++ APIs.
#2. What is the call stack
A call stack is a mechanism for an interpreter to keep track of its place in a script that calls multiple functions. If you have a code file that has 5 functions called, it will keep track of which function has been called and will be called next. Imagine that to be like a pile of cards. When you call a function, you put a card onto the top of the pile. When another function is called, then another card. The top most card is the function the interpreter is currently executing.
Once the function is completed, it will be removed from the top of the pile until there is none left.
Source from wikihow
In a slightly more technical term, the stack keeps track of execution contexts.
When developers mention that JavaScript is a single thread language. What single threaded means is it only has one call stack and can only do one thing at any one time. When a function is called, it is added on top of a stack like a stack of cards. When the function completes, it will be removed from the call stack from the top.
One thread == one call stack == one thing at a time
Here’s an example of an actual call stack:
- Baz is called. Adding a call named baz to the empty pile of cards.
- In function Baz, we called bar. Adding a card named bar on top of baz.
- bar called foo, Adding a card named foo on top of bar.
- foo threw an error, and this entire stack was returned to the browser and printed on the console log.
The bottom most card: anonymous function is basically your main function that the application has to run to even start calling baz (the first function call)
What happens when a function calls itself? When will it end?
When a function ends up in a recursive loop, like a function calling itself. And it looks just like this. It goes on and on.
But all good things must come to an end. So when the recursive function goes on, you will hit a hard dead end; the limit of the stack. Then you will get an error call the Maximum call stack size exceeded. In different JavaScript environments, there are different limits:
- Node.js: 11034
- Firefox: 50994
- Chrome: 10402
Cool facts thus far? Nothing works magically and when you decompose an application you can see its gears putting everything together like a master piece. Found a little cartoon, share with you.
credit: theburningmonk
#3. What is event loop?
The Event Loop has one job — to monitor the Call Stack and the Callback Queue. If the Call Stack is empty, it will take the first event from the queue and push it to the Call Stack, and effectively runs it.
What happens when things are slow? Anything that is slow on the stack are blocking
I love how this speaker talks about these functions with relevant context of usage. In Javascript, it is every common to use this tool called Promises and asynchronous functions. There are just so much we can learn from these two concepts alone so we will not go into through right now. I may even consider doing a different article just about them. Until then, here’s a guide about promises I find really insightful. Check it out here.
Here’s an example:
When we do a setTimeout, and you can check out the setTimeout function here:
- It first goes to the stack.
- Then it goes to the webapi. It is sent to the WebAPI because the V8 engine does not have the setTimeout function.
- Then once the timeout is cleared, it will use your callback function to the task queue
- Then the event loop will check both the stack and task queue.
- If the stack is empty, it will take the first thing on the task queue and push it to the stack.
I’ve found a really interesting site that can actually help us to visualize the entire code. You can click here to check out the code. One simple click of a button and there are so many steps that are executed eloquently for you under the hoop.
So for the engineers out there, what is the difference between immediate execution of a function as compared to putting a setTimeout, t=0 to execute the function?
Here’s the answer: When you put setTimeout with time = 0, it doesn’t actually get executed immediately. Instead, it will wait until the stack is cleared before that event gets called.
From an asynchronous function is called, that function actually leaves the call stack, and thus needs to be requeued back into the stack, and that is the role of the event loop. Once again:
The Event Loop has one job — to monitor the Call Stack and the Callback Queue. If the Call Stack is empty, it will take the first event from the queue and push it to the Call Stack, and effectively runs it.
Another fun fact: setTimeout does not set the time delayed before the function gets executed. Rather, it is the minimum time before the function gets called.
Lets check out what is happening visually. You can check out the code here.
Just to end of the article with a brief touch-and-go about web browser events - in this example we will use the form of a button click.
When you do a button click, it does not get pushed into the stack immediately. Instead, when you click, there is a click even that is executed by the Web APIs, then that function will get pushed into the callback queue, and it will be pushed into the stack by the event loop when the call stack is empty. This is the original page with a set of code that allows you to click the button and find out the sequence of events that happened upon that click. You can check it out in this url
TLDR;
So when people mention not to block the event loop, that means don’t put slow blocking code on the call stack and thus the event loop cannot do anything before the call stack gets cleared out.
Neither do you want to flood the callback queue, thats when you can consider using debounce to only queue one slow function call every X millisecond to avoid flooding the callback queue.
Even after all is said and done, this is merely just the tip of the iceberg to the JavaScript engine and environment. The V8 engine has so many capabilities including garbage collection, translator and interpreter. We can find out more about this topic here.
Reference: